我們在開發專案的時候,為了程式碼的品質 通常會用各種輔助工具幫助我們檢查(例如: ESLint, Formater, Testing …)
Git hooks 可以透過在 before commit 之前做一些檢查,雖然會稍微慢一點 但是為了整體的開發格式是 OK 的
此文件主要是在討論 一種情況
今天你可能在開發 Vue 元件的時候,你可能不希望有一些元件被引用了不該引用的檔案或是使用套件
EX:
填寫表單的頁面 不允許使用 Pinia or 使用特定的元件( 避免相依性之類)
這時候除了寫文件以外 一定都會想到 那我就在註解 OR Commit 的時候檢查,這時候 EsLint Local Rules 就出現了
PreRequires
Vue & Vite Cli
主要是以下的套件需要安裝:
可以參考從Husky Lint-staged 開始安裝
- Husky install
此套件主要是可以比較簡單操作 Git hooks
1 | npx husky-init && npm install |
- Lint staged install
此套件主要是針對 Commit Code 只會針對這些 Commit 的檔案做優化 以及檢查 而不會所有專案內的檔案檢查 增加效率
1 | npm i --save-dev lint-staged |
- Modify .husky pre-commit file
修改.husky 資料夾底下的 pre-commit 檔案
1 | #!/bin/sh |
- Modify package.json
修改 package.json 直接在 root 底下新增這個 KEY
可以參考官方文件 也可以分離出來Config
我們只需要針對 vue ,ts, js 檔案做優化
1 | "lint-staged": { |
接著就可以隨便測試看看 Commit 一個不合法的 js 檔案
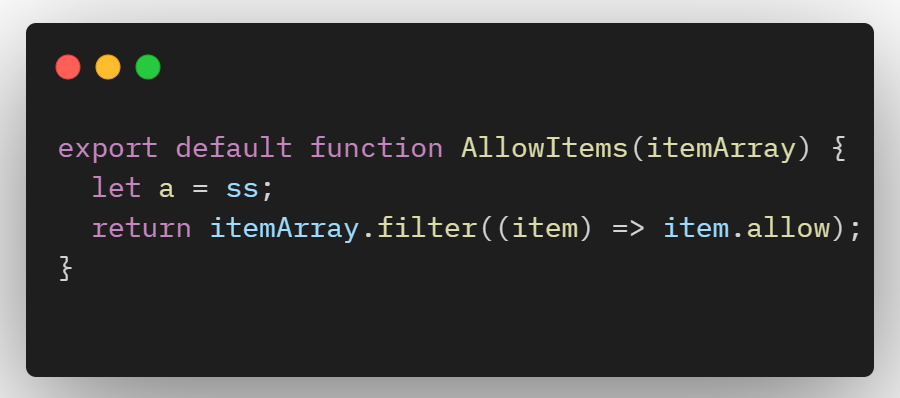
正常會在 GIT Console 上面顯示 eslint 檢查出來的錯誤 這樣就設定完成了
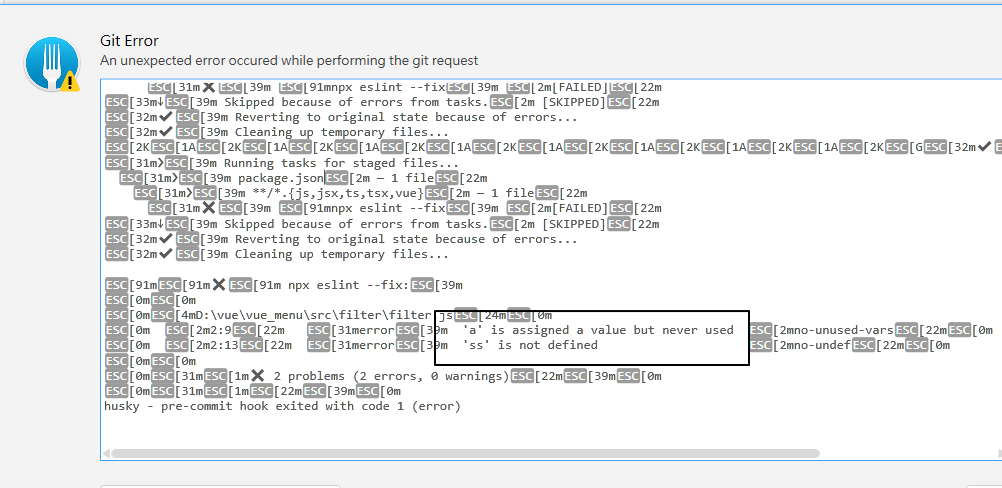
EsLint Local Rules
接著介紹今天的主角,如果我們想要客製一些 只有我們專案會用到的規則
就可以透過eslint-plugin-local-rules來達到
這裡直接跳過安裝,從設定開始
Setting EsLint Local Rules
- 在你的 ESLint Config 設定 plugin &你想要綁定的 rule 以及錯誤的程度
以 vue cli 來說預設是在 package.json 裡面 的 rules Object
新增你自訂的規則以及 plugin
1 | "eslintConfig": { |
- 接著 create eslint-local-rules folder and index.js 制定出我們需要的規則
文件可以參考此working-with-rules
首先我們先以Demo
來看
我們先自訂一個 local rules 用途主要是 comment 有
//eslint-disable-package: testbutton
他就去檢查 import 的元件是否有 TestButton 這個字眼
1 | module.exports = { |
我們可以透過 Commit 來測試,也可以透過 report 錯誤把資訊印出來 Debug
1 | context.report({ |
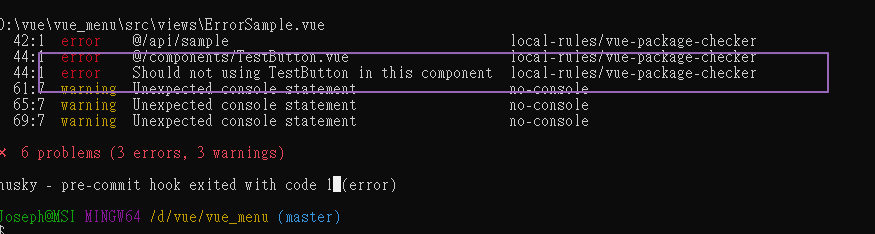
在上面的連結中 也有各式各樣的 Statement 可以去檢查變數的命名或是 Catch 的錯誤之類的資訊
VariableDeclaration
CatchClause
也可以參考以下這篇文章
中文版 Custom Rules
….
用途
這個用途主要是在 如果在測試不足的狀況下
可以透過自訂 ESLINT 規則來規範團隊的開發.並且避免一些不必要的引用. 尤其是有可能這個元件也會需要給第三方 或是其他人使用
Reference
Commit Better Code with Husky, Prettier, ESLint, and Lint-Staged
Husky - Git Hooks 工具
working-with-rules